How to use AWS Bedrock to Analyze BIM Data
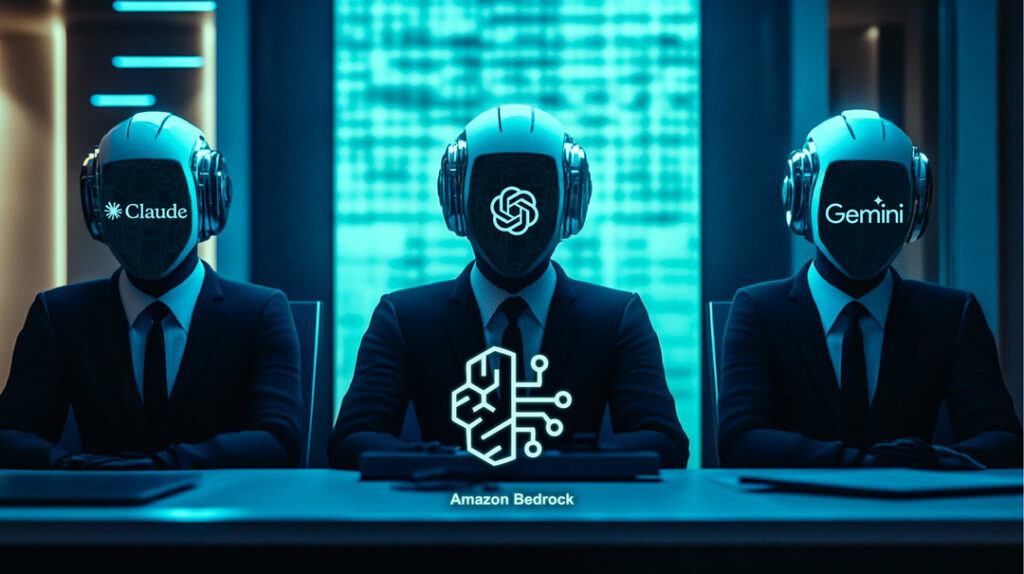
By now, we’re all familiar with the immense benefits of AI—and more specifically, Large Language Models (LLMs).
We’ve talked countless times about how they’re revolutionizing automation, data processing, and even 3D modeling.
The pace of innovation is breathtaking. New players like DeepSeek are emerging, while trusted giants like ChatGPT, Claude, and Gemini continue to roll out powerful new versions.
This rapid evolution is a double-edged sword:
While it’s exciting to see better models released so frequently, it becomes a real challenge when your product is tightly coupled to a specific model. Migrating to a new one can feel like rebuilding your stack every few months.
Fortunately, there’s a solution—AWS offers a service that takes this headache off your plate.
It lets you connect your application to a unified endpoint, and behind the scenes, you can swap out the underlying model with ease. No refactoring, no disruption—just flexibility.
Let’s dive into how it works and how it can future-proof your AI-powered products.
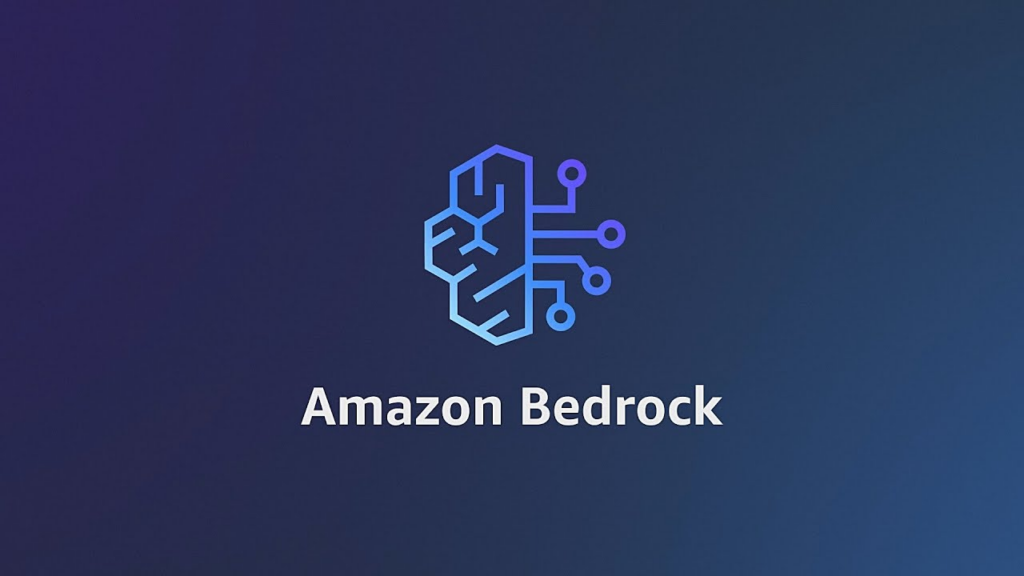
1. Understanding AWS Bedrock
AWS Bedrock is a fully managed service that gives you API-based access to a variety of foundation models (FMs) from leading AI companies—all without the need to manage any infrastructure. It acts as a unified gateway to more than 150 models from providers like:
- Anthropic (Claude family – ideal for conversations, reasoning, summarization)
- AI21 Labs (Jurassic models – strong at long-form generation and rich text tasks)
- Cohere (Command R+ and others – optimized for retrieval-augmented generation and enterprise apps)
- Meta (Llama models – open-weight models with broad general-purpose capabilities)
- Amazon Titan (Amazon’s own FMs – for text and embeddings tasks)
With Bedrock, you can test, compare, and deploy multiple models using a single API structure, which makes switching between models incredibly easy.
Whether you’re building a chatbot, analyzing documents, generating code, or creating intelligent search tools, Bedrock lets you adapt quickly to the latest and best-performing models without locking into a single vendor.
This is especially valuable in fast-moving fields like generative AI, where new models can leapfrog existing ones in a matter of weeks.
Bedrock future-proofs your development by allowing model swapping with minimal changes to your application code, and it supports fine-tuning and agents, so you can build more personalized and autonomous systems on top.
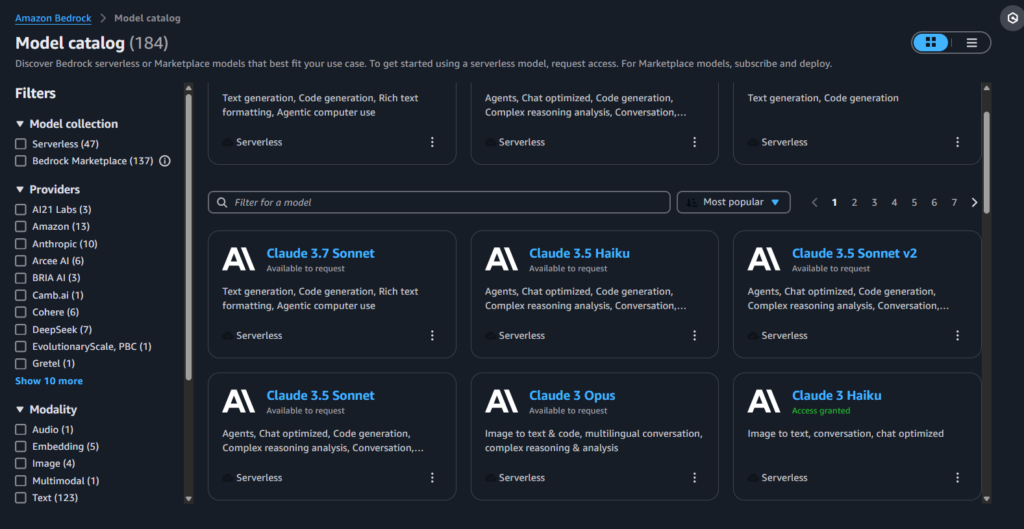
2. Setting Up AWS Bedrock
Step 1: Enable AWS Bedrock
- Sign in to your AWS account.
- Navigate to AWS Bedrock in the AWS Console.
- Enable the foundation models you want to use (e.g., Anthropic Claude, AI21 Jurassic, Amazon Titan).
- Set up IAM permissions to allow API access to AWS Bedrock.
Step B: Create an IAM Role for Bedrock
You’ll need an IAM role with the following permissions:
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Action": [
"bedrock:InvokeModel",
"bedrock:ListFoundationModels"
],
"Resource": "*"
}
]
}
Attach this policy to your AWS Lambda or API Gateway.
3. Switching Between AI Models in Bedrock
To optimize querying and automation, we can switch AI models based on use case:
- Anthropic Claude → Best for complex natural language understanding, summarization, and reasoning.
- AI21 Jurassic → Useful for structured text generation, such as generating schedules or automating documentation.
- Amazon Titan → General-purpose NLP for data extraction and classification.
Code Example: Choosing an AI Model Dynamically
With something like the code below you could actually even present multiple answers from multiple models and let the user decide which model to use.
import boto3
import json
# AWS Bedrock client
bedrock = boto3.client('bedrock-runtime', region_name='us-east-1')
def invoke_model(model_id, prompt):
response = bedrock.invoke_model(
body=json.dumps({
"prompt": prompt,
"max_tokens_to_sample": 500
}),
modelId=model_id
)
return json.loads(response['body'].read().decode('utf-8'))
# Define use cases
models = {
"querying": "anthropic.claude-v2",
"automation": "ai21.j2-ultra",
"classification": "amazon.titan-text"
}
# Example usage
user_prompt = "Extract all wall elements and their materials from the Revit model."
selected_model = models["querying"]
response = invoke_model(selected_model, user_prompt)
print(response)
4. Connecting AI Models to Revit API
We can use a serverless function (AWS Lambda or Flask/FastAPI) to connect the AI model to a Revit plugin, like the example of the AI Assistant that we did.
Step 1: Creating a Revit Add-in (C#)
Create a basic Revit add-in that sends BIM queries to AWS Bedrock.
public class RevitAIQuery : IExternalCommand
{
public Result Execute(ExternalCommandData commandData, ref string message, ElementSet elements)
{
Document doc = commandData.Application.ActiveUIDocument.Document;
// Extract wall data
FilteredElementCollector collector = new FilteredElementCollector(doc);
var walls = collector.OfClass(typeof(Wall)).ToElements();
List wallInfo = new List();
foreach (Wall wall in walls)
{
wallInfo.Add($"Wall ID: {wall.Id}, Material: {wall.Name}");
}
// Send data to AWS Lambda API
string apiEndpoint = "https://your-api-gateway.amazonaws.com/prod/query";
HttpClient client = new HttpClient();
var content = new StringContent(JsonConvert.SerializeObject(wallInfo), Encoding.UTF8, "application/json");
var response = client.PostAsync(apiEndpoint, content).Result;
TaskDialog.Show("AWS Bedrock Response", response.Content.ReadAsStringAsync().Result);
return Result.Succeeded;
}
}
5. Deploying an AWS Lambda Function for AI Processing
AWS Lambda will receive the request, send it to AWS Bedrock, and return the AI-processed result.
Python Code for AWS Lambda
import json
import boto3
bedrock = boto3.client('bedrock-runtime', region_name='us-east-1')
def lambda_handler(event, context):
# Extract data from Revit request
body = json.loads(event["body"])
walls = body["walls"]
# AI model selection
model_id = "anthropic.claude-v2"
# Construct prompt
prompt = f"Analyze these walls and suggest improvements: {walls}"
# Invoke AWS Bedrock
response = bedrock.invoke_model(
body=json.dumps({"prompt": prompt, "max_tokens_to_sample": 300}),
modelId=model_id
)
result = json.loads(response['body'].read().decode('utf-8'))
return {
"statusCode": 200,
"body": json.dumps(result)
}
6. Automating Revit Workflows Using AI
One of the most powerful applications of LLMs in the AEC industry is their ability to automate tedious, manual Revit tasks—especially when paired with structured BIM data and Revit’s robust API. Below are some key use cases, now with more implementation insight and real-world relevance.
Use Case 1: Auto-Classifying Revit Elements
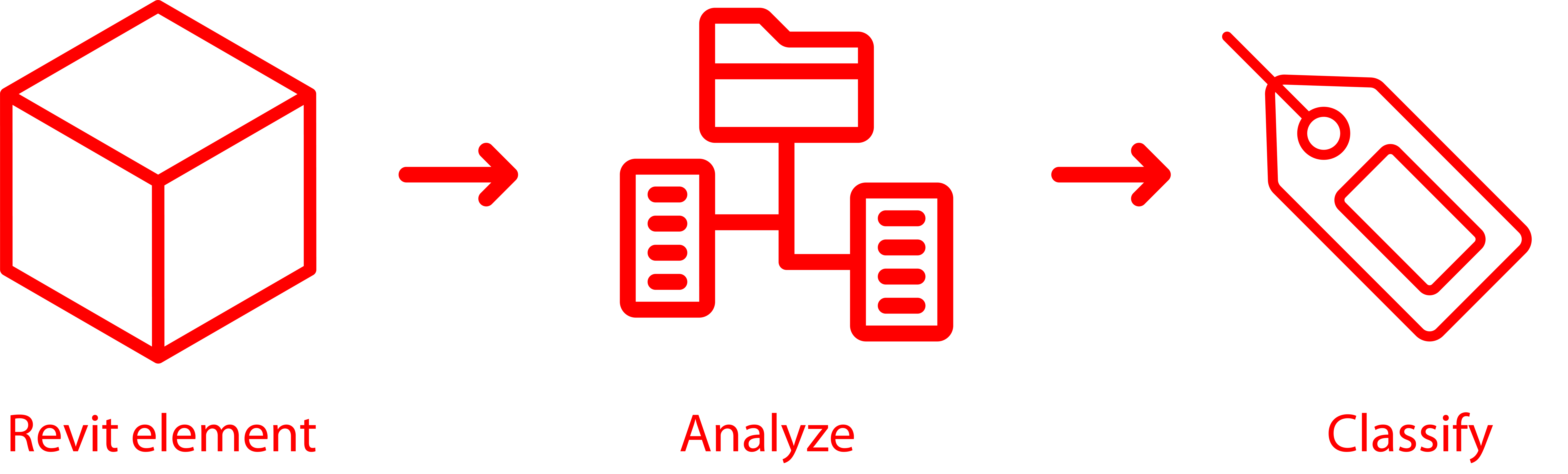
Problem:
Tagging and classifying Revit elements manually is time-consuming and error-prone, especially in large models with hundreds or thousands of components.
Solution:
You can send metadata like element name, type, dimensions, and location to an AI model via AWS Bedrock. The model returns a classification or category label based on natural language patterns or custom rules.
Example Prompt:
“Given the following element data, classify the element into architectural, structural, MEP, or furniture categories. Data: {element_type: ‘FamilyInstance’, name: ‘Duct Terminal’, level: ‘Level 3’, material: ‘Galvanized Steel’}”
Possible Output:
“Category: MEP – HVAC”
I know this categorization might seem obvious but you can filter by much more complex plogic and then write Revit API code to automatically assign this classification to a shared parameter or tag in the model.
Use Case 2: Optimizing Materials & Energy Analysis
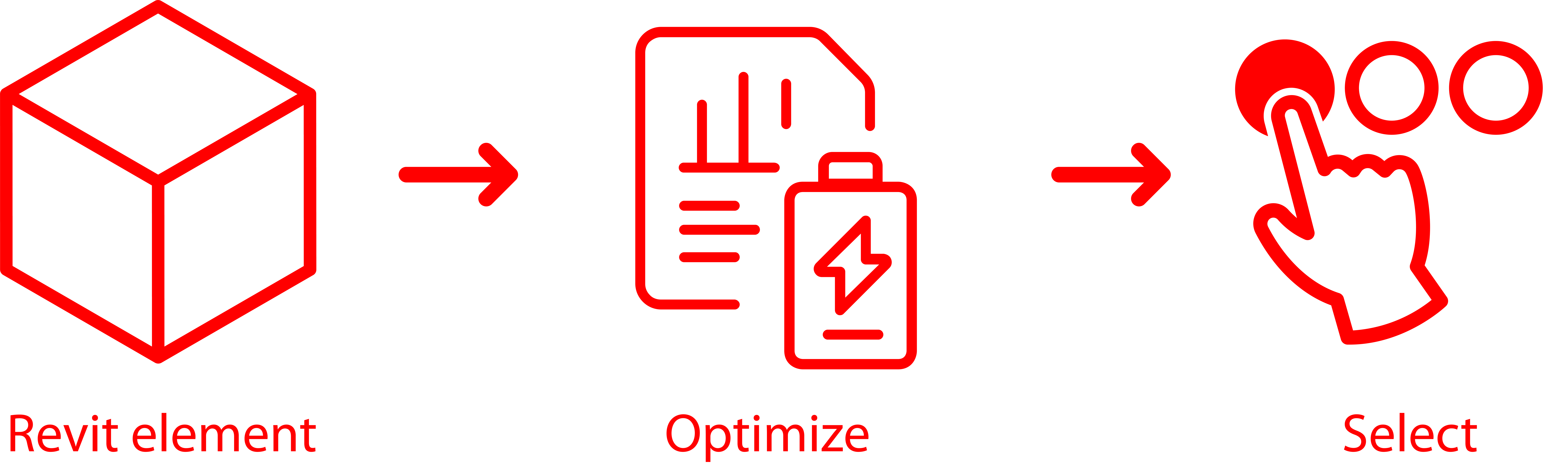
Problem:
Many designers don’t have time or tools to assess the energy efficiency or sustainability of materials during early design phases.
Solution:
Using AWS Bedrock and foundation models with sustainability expertise (e.g., Titan or Claude), you can analyze existing wall, floor, and roof materials and recommend greener alternatives.
Implementation Flow:
- Revit Add-in exports materials used in walls/floors.
- Lambda sends this to an LLM with a prompt like:
“For the following wall materials, suggest greener alternatives with lower embodied carbon. Include thermal performance and cost implications.”
- Bedrock model returns suggestions like:
“Replace ‘Concrete – 3000 psi’ with ‘Hempcrete’ for a 35% reduction in embodied carbon and improved insulation.”
- The suggestion is displayed in Revit or even logged for reporting.
This supports LEED certification efforts or early-stage sustainability goals.
Use Case 3: Natural Language Querying for BIM Data
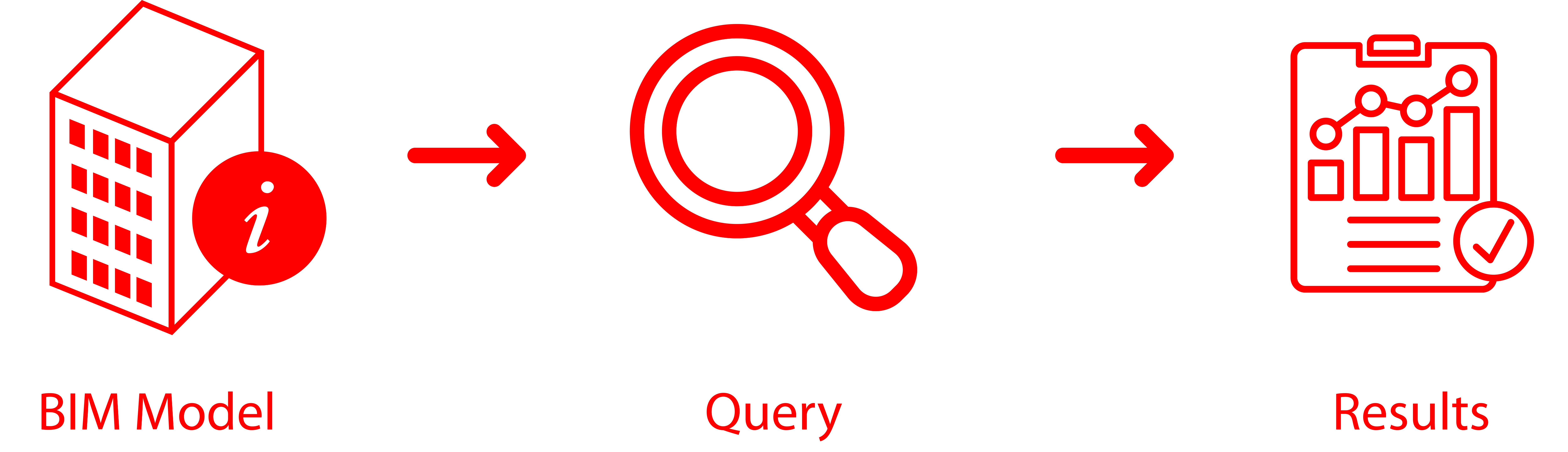
Problem:
Users without programming or Dynamo knowledge struggle to extract insights from BIM models.
Solution:
Allow users to ask questions in plain English, which are parsed and translated into data queries by an LLM.
Example Queries:
- “How many concrete walls are in the model?”
- “List all HVAC components above the second floor.”
- “What is the total window area on the south facade?”
Behind the scenes:
- The prompt is sent to AWS Bedrock.
- The LLM interprets the intent and returns a pseudo-query or filter conditions.
- Your Lambda or add-in parses that and uses Revit API to fetch results.
- Response is shown in a user-friendly format (table, report, tooltip, etc.).
Bonus: You can also log these queries to identify what insights your users care most about—and then automate those queries.
AWS Bedrock: much more than an integration
The AEC industry is on the cusp of a transformation—where design decisions, model insights, and routine tasks can be augmented or fully automated through the power of generative AI.
By integrating AWS Bedrock into your tech stack, you gain the flexibility to leverage the best models for each task, dynamically switch between providers like Anthropic, AI21, and Amazon Titan, and avoid the lock-in that often plagues AI-powered products.
This architecture doesn’t just improve productivity—it reshapes how architects, engineers, and BIM managers interact with their data:
- Revit models become queryable through natural language.
- AI can classify, optimize, and even recommend design improvements in real time.
- Teams can respond faster to RFIs, compliance checks, and sustainability goals.
Most importantly, you don’t have to rebuild your stack when a better model comes out next quarter—you just switch the model behind the same API.
This is how you scale AI in AEC: with a modular, future-proof foundation that connects cloud intelligence to the tools your teams already use.
Valentin Noves
I'm a versatile leader with broad exposure to projects and procedures and an in-depth understanding of technology services/product development. I have a tremendous passion for working in teams driven to provide remarkable software development services that disrupt the status quo. I am a creative problem solver who is equally comfortable rolling up my sleeves or leading teams with a make-it-happen attitude.